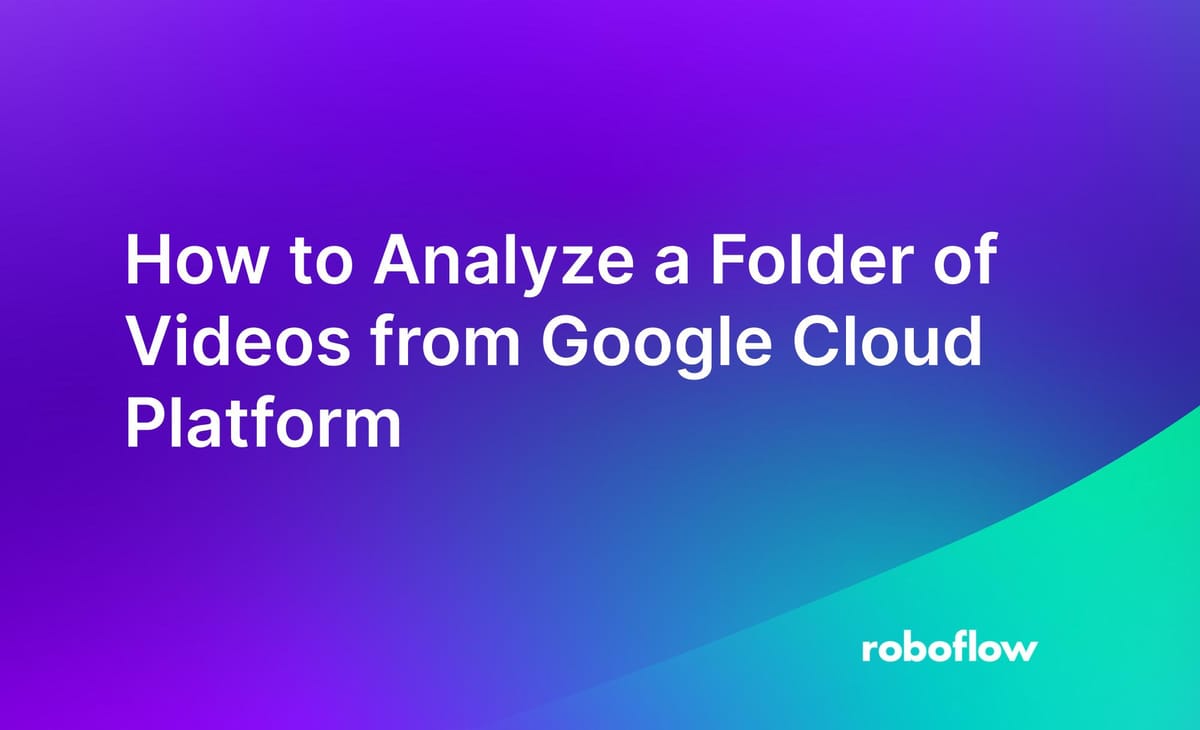
Utilizing laptop imaginative and prescient, you possibly can analyze movies to uncover insights related to your venture.
For instance, you possibly can use a soccer participant detection mannequin on a folder of photos saved within the cloud to calculate statistics a couple of recreation or use a basis mannequin, like CLIP, to categorise frames in a video and to establish when particular scenes (i.e. outside scenes) occur in a video.
Utilizing the Roboflow Video Inference API, you possibly can analyze movies utilizing fine-tuned laptop imaginative and prescient fashions (i.e. a soccer participant detection mannequin, a crack detection mannequin) and state-of-the-art basis imaginative and prescient fashions equivalent to CLIP.
On this information, we’re going to present learn how to analyze a folder of movies hosted on Google Cloud Platform with the Roboflow Video Inference API.
By the tip of this information, we may have the next video that reveals the outcomes from operating an object detection mannequin on a soccer recreation:
With out additional ado, let’s get began!
Step #1: Choose a Pc Imaginative and prescient Mannequin
You should use the Roboflow Video Inference API with:
- Public fine-tuned imaginative and prescient fashions hosted on Roboflow Universe;
- Fashions you will have privately educated on Roboflow, and;
- Basis fashions equivalent to CLIP and a gaze detection mannequin.
Roboflow Universe hosts over 50,000 pre-trained fashions that cowl a variety of use circumstances, from logistics to sports activities evaluation to defect detection. You probably have a use case in thoughts, there could already be a Universe mannequin you need to use.
If you’re constructing a logistics software, for instance, we advocate the Roboflow Universe Logistics mannequin. This mannequin can establish 20 totally different objects related to logistics equivalent to picket pallets and folks or you need to use it as pre-trained weights which might carry out higher than COCO on logistics use circumstances.
You should use a mannequin you will have educated on Roboflow to research a video, too. For instance, when you’ve got educated a defect detection mannequin by yourself information, you need to use that video to research your movies. To be taught extra about learn how to practice your individual laptop imaginative and prescient mannequin, check with the Roboflow Getting Began information.
You can too use basis fashions hosted on Roboflow. For instance, you need to use CLIP to categorise frames in a video, supreme for video evaluation. Or you need to use our gaze detection system to establish the path wherein individuals are wanting, supreme for examination proctoring. Learn the Roboflow Video Inference API documentation to be taught extra about supported basis fashions.
Step #2: Submit Movies for Evaluation
Upon getting chosen a mannequin, you’re prepared to start out submitting movies from Google Cloud Platform for evaluation. We’re going to use a soccer participant detection mannequin for this tutorial, however you need to use any mannequin chosen in response to the final part.
First, we have to set up a couple of dependencies:
pip set up google-cloud-storage roboflow supervision
We are going to use google-cloud-storage
to retrieve our movies from GCP Cloud Storage. We are going to use the roboflow
bundle to submit movies for evaluation. We are going to use supervision
to plot predictions from the Roboflow Video Inference API onto a video.
Subsequent, we have to authenticate with Google Cloud. We will accomplish that utilizing the gcloud
CLI. To learn to authenticate with Google Cloud, check with the official Google Cloud authentication documentation.
Additionally, you will want a service account with permission to learn the contents of your GCP Storage buckets. Consult with the IAM documentation for extra info. When you will have created your service account, obtain a JSON key from the IAM dashboard on your service account.
We are actually prepared to write down a script to submit movies for evaluation.
Create a brand new Python file and add the next code:
from google.cloud import storage
import datetime
import tqdm
from roboflow import Roboflow BUCKET_NAME = "my-bucket" rf = Roboflow(api_key="API_KEY")
venture = rf.workspace().venture("football-players-detection-3zvbc")
mannequin = venture.model(2).mannequin storage_client = storage.Consumer.from_service_account_json("./credentials.json")
bucket = storage_client.bucket(BUCKET_NAME) information = [file.name for file in bucket.list_blobs()] file_results = {ok: None for ok in information} for file in tqdm.tqdm(information, complete=len(information)):
url = bucket.blob(file).generate_signed_url(
model="v4",
expiration=datetime.timedelta(minutes=2),
technique="GET",
) job_id, signed_url = mannequin.predict_video(
url,
fps=5,
prediction_type="batch-video",
) outcomes = mannequin.poll_until_video_results(job_id) file_results[file] = outcomes with open("outcomes.json", "w+") as results_file:
results_file.write(str(outcomes))
On this code, we:
- Make a listing of all information within the specified GCP bucket.
- Ship every file for evaluation to the Roboflow API utilizing the desired mannequin. On this instance, now we have used a soccer participant detection mannequin.
- Look ahead to outcomes from every evaluation utilizing the
poll_until_video_results
operate. - Save the outcomes from inference to a JSON file.
Substitute credentials.json
with the IAM JSON credentials file on your service account.
The poll_until_video_results
operate waits for outcomes to be out there. The operate is obstructing. For big-scale use circumstances, you possibly can adapt the code above to assist concurrency so you possibly can start a number of analyses on the similar time with out ready for the outcomes from each.
Within the code above, substitute:
MODEL_ID
andVERSION
with the mannequin ID and model ID related along with your Roboflow mannequin. Discover ways to retrieve your Roboflow mannequin and model IDs.API_KEY
along with your Roboflow API key. Discover ways to retrieve your Roboflow API key.fps=5
with the FPS at which you wish to run movies. With `fps=5`, inference is run at a price of 5 frames per second. We advocate selecting a low FPS for many use circumstances; selecting a excessive FPS will end in the next evaluation value since extra inferences have to be run.
Then, run the code.
The code above saves outcomes to a file known as outcomes.json
. The outcomes from every inference are related to the title of the file that was processed. The file takes the next construction:
{“file.mp4”: inference_results}
To be taught in regards to the construction of inference_results
, check with the Roboflow Video Inference API documentation for the mannequin you’re utilizing. For this information, we are able to check with the fine-tuned mannequin documentation since we’re working with an fine-tuned object detection mannequin.
Step #3: Visualize Mannequin Outcomes
Within the final step, we ran evaluation on information in a Google Cloud bucket. Now, let’s visualize the mannequin outcomes. For this part, we’ll give attention to exhibiting learn how to visualize object detection outcomes since this information walks by an object detection use case.
Create a brand new Python file and add the next code:
import supervision as sv
import numpy as np
import json
import roboflow roboflow.login() rf = roboflow.Roboflow()
venture = rf.workspace().venture("football-players-detection-3zvbc")
mannequin = venture.model(2).mannequin VIDEO_NAME = "video1.mp4"
MODEL_NAME = "football-players-detection-3zvbc" with open("outcomes.json", "r") as f: outcomes = json.load(f) model_results = outcomes[VIDEO_NAME][MODEL_NAME] for end in model_results: for r in end result["predictions"]: del r["tracker_id"] frame_offset = outcomes[VIDEO_NAME]["frame_offset"] def callback(scene: np.ndarray, index: int) -> np.ndarray: if index in frame_offset: detections = sv.Detections.from_roboflow( model_results[frame_offset.index(index)] ) class_names = [i["class"] for i in model_results[frame_offset.index(index)]["predictions"]] else: nearest = min(frame_offset, key=lambda x: abs(x - index)) detections = sv.Detections.from_roboflow( model_results[frame_offset.index(nearest)] ) class_names = [i["class"] for i in model_results[frame_offset.index(nearest)]["predictions"]] bounding_box_annotator = sv.BoundingBoxAnnotator() label_annotator = sv.LabelAnnotator() labels = [class_names[i] for i, _ in enumerate(detections)] annotated_image = bounding_box_annotator.annotate( scene=scene, detections=detections) annotated_image = label_annotator.annotate( scene=annotated_image, detections=detections, labels=labels) return annotated_image sv.process_video( source_path="video.mp4", target_path="output.mp4", callback=callback,
)
On this code, we obtain a video from the Google Cloud Storage API. We then retrieve the inference outcomes for that video that we calculated within the final step. We open the uncooked video, plot inference outcomes on every body, then save the outcomes to a brand new file known as “output.mp4”.
Within the final step, we ran inference at 5 FPS. Which means we don’t have outcomes to plot for a lot of frames. This might create a flickering impact since we run inference at 5 FPS and our video is saved at 24 FPS.
To counter this, the script above chooses the outcomes closest to the present body. Thus, if there aren’t any predictions for a body, our code will plot predictions from the closest body.
If you’re utilizing a segmentation mannequin, substitute sv.BoundingBoxAnnotator()
with sv.MaskAnnotator()
to visualise segmentation outcomes.
Let’s run our code. Listed here are the outcomes of predictions plotted on a video:
We’ve efficiently plotted predictions from the Roboflow Video Inference API on our video.
The supervision
library we used to plot predictions has a variety of utilities to be used in working with object detection fashions. For instance, you possibly can filter predictions by confidence, depend predictions in a specified zone, and extra. To be taught extra about constructing laptop imaginative and prescient functions with `supervision`, check with the supervision documentation.
Conclusion
You should use the Roboflow Video Inference API to run laptop imaginative and prescient fashions on movies saved in Google Cloud Storage. On this information, we demonstrated learn how to analyze movies saved in Google Cloud Storage utilizing a public laptop imaginative and prescient mannequin hosted on Roboflow Universe.
First, we authenticated with Google Cloud Storage. Then, we generated signed URLs for every video in a bucket and despatched them to the Roboflow Video Evaluation API to be used in video evaluation. We requested that the Roboflow Video Inference API run a soccer participant detection mannequin on our video. You’ll be able to analyze movies with fashions on Roboflow Universe, fashions you will have educated privately on Roboflow, or utilizing basis fashions equivalent to CLIP.