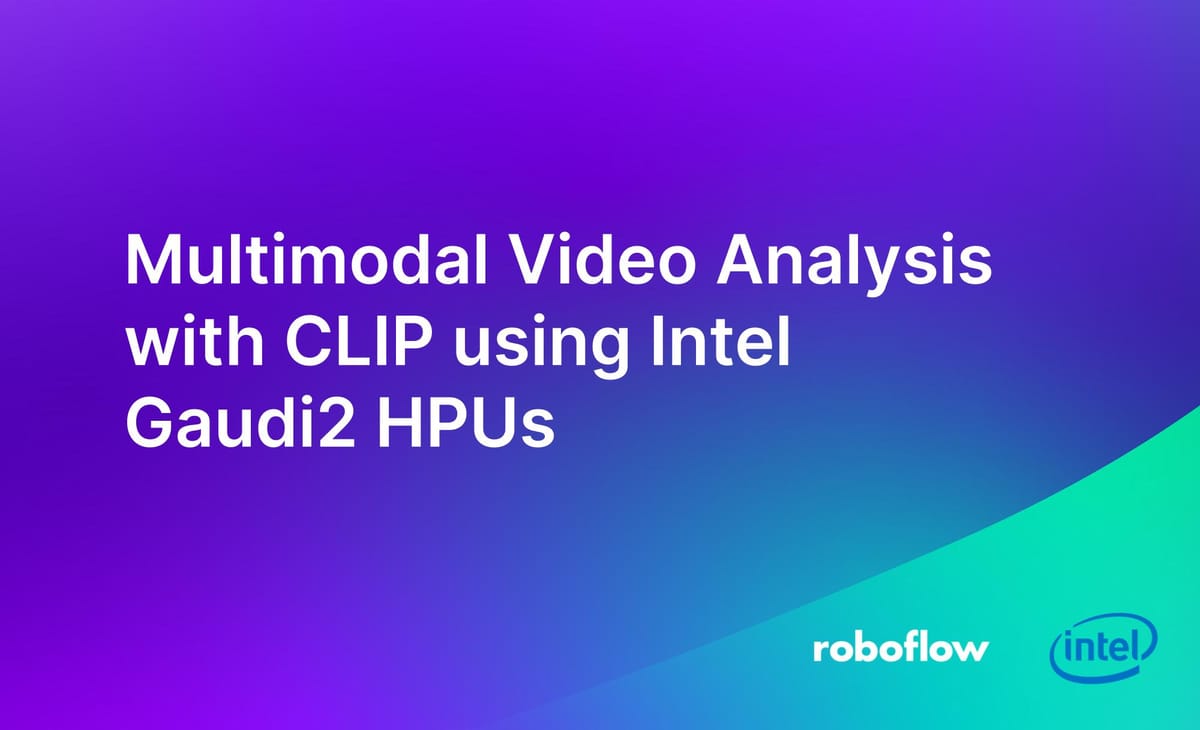
You should use the multimodal mannequin CLIP to categorise frames in a video. That is helpful for media indexing use instances the place you wish to assign labels to photographs. You can assign a single label to a video (i.e. whether or not a video does or doesn’t comprise a violent scene) or a number of labels (i.e. a video accommodates an workplace scene, a park scene, and extra).
On this information, we’re going to classify the frames in a video for knowledge use in knowledge analytics utilizing CLIP, an open supply multimodal mode by OpenAl, and the Gaudi2 system. Gaudi2 is developed by Habana, an Intel firm. We’re going to use this technique to judge whether or not a video accommodates varied scene descriptors. We’ll seek for a park scene and an workplace scene in a video.
The Gaudi2 system is designed for big scale purposes, providing 96 GB HBM2E reminiscence and twin matrix multiplication engines in every chip. You can use the steering on this tutorial to scale as much as processing hundreds of movies.
With out additional ado, let’s get began!
What’s CLIP?
Contrastive Language Picture Pre-training (CLIP) is a multimodal laptop imaginative and prescient mannequin developed by OpenAI. With CLIP, you’ll be able to examine the similarity of two photos, or the similarity of a picture to a collection of textual content labels. You should use the latter performance to categorise video frames.
To make use of CLIP for video classification, we are going to:
- Set up CLIP.
- Calculate CLIP vectors for each body in a video.
- Determine essentially the most comparable class to every body.
- Assign tags to timestamps within the video.
Step #1: Set up Dependencies
For this tutorial, we’re going to set up the Transformers implementation of CLIP. We will specify that we wish to use our Gaudi2 chip, which is optimized for machine studying workloads, to run CLIP with the Transformers CLIP implementation.
To put in Transformers, run the next command
pip set up transformers
To course of our video, we’re going to use the supervision Python package deal. This package deal accommodates a variety of utilities to be used in constructing laptop imaginative and prescient purposes. We’ll use the supervision video functionalities to divide a video into frames. We’ll then classify every body with CLIP.
To put in supervision, run:
pip set up supervision
We at the moment are prepared to begin writing the logic for our software.
Step #2: Calculate CLIP Vectors for Video Frames
Let’s classify the trailer of the film Contact. This trailer options scenes that embrace computer systems, workplaces, satellites, and extra.
Earlier than we are able to classify every body, we have to compute CLIP vectors for every body. As soon as we have now these embeddings, we are able to examine textual content embeddings from labels (i.e. “laptop”, “park”, “satellite tv for pc”) to every body to establish which label most precisely represents every body.
Let’s begin by importing the required dependencies and initializing a couple of variables we are going to use in our script:
attempt: import habana_frameworks.torch.core as htcore DEVICE = "hpu"
besides ImportError: DEVICE = "cpu" from transformers import CLIPProcessor, CLIPModel
import supervision as sv
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity mannequin = CLIPModel.from_pretrained("openai/clip-vit-base-patch32").to(DEVICE)
processor = CLIPProcessor.from_pretrained("openai/clip-vit-base-patch32") VIDEO = "trailer.mp4" PROMPTS = ["satellite", "office", "park", "computer", "outdoors", "meetings", "something else"]
INTERVAL_PERIOD = 5
outcomes = []
Within the code above, we:
- Import the required dependencies
- Load the CLIP mannequin and solid it to our HPU chip
- Declare variables we are going to use all through our script
Within the script, exchange:
VIDEO
with the title of the video you wish to analyze.PROMPTS
with the prompts you wish to use in video classification.INTERVAL_PERIOD
with the interval (in seconds) you wish to use to investigate your video by timestamp. A 5 worth signifies that we’ll generate a report that reveals the most typical immediate each 5 seconds in our video afterward on this information.
CLIP works with an open vocabulary. This implies there isn’t any “grasp record” of prompts that you may specify. Moderately, you’ll be able to specify any label you need. With that stated, we suggest testing completely different labels to see which of them are simplest in your use case.
On this information, we use the prompts:
- satellite tv for pc
- workplace
- park
- laptop
- outside
- conferences
- one thing else
“one thing else” is an efficient null class. If not one of the specified labels match, “one thing else” is extra prone to match.
Subsequent, we have to declare a couple of capabilities to assist calculate embeddings. We’ll use embeddings to investigate the contents of our video.
def get_image_embedding(picture): inputs = processor(photos=[image], return_tensors="pt", padding=True).to(DEVICE) outputs = mannequin.get_image_features(**inputs) return outputs.cpu().detach().numpy() def get_text_embedding(textual content): inputs = processor(textual content=[text], return_tensors="pt", padding=True).to(DEVICE) outputs = mannequin.get_text_features(**inputs) return outputs.cpu().detach().numpy() def classify_image(picture, prompts): image_embedding = get_image_embedding(picture) sims = [] for immediate in prompts: prompt_embedding = PROMPT_EMBEDDINGS[prompt] sim = cosine_similarity(image_embedding, prompt_embedding) sims.append(sim) return PROMPTS[np.argmax(sims)]
Within the code above, we declare three capabilities: one to calculate picture embeddings, one to calculate textual content embeddings, and one which makes use of all our textual content embeddings and a single picture embedding to return a single label for a body.
Now, let’s analyze our video!
Video Evaluation with CLIP
For every body in our video, we wish to discover essentially the most related label. We will do that utilizing the next algorithm:
- Calculate textual content embeddings for all our prompts and save them for later use.
- Take a video body.
- Calculate a picture embedding for the video body.
- Discover the textual content embedding that’s most just like the video body.
- Use the label related to that textual content embedding as a label for the body.
We will repeat this course of for every body in our video to categorise video frames.
Add the next code to the Python script we began within the final step:
PROMPT_EMBEDDINGS = {immediate: get_text_embedding(immediate) for immediate in PROMPTS} for i, body in enumerate(sv.get_video_frames_generator(source_path=VIDEO, stride=1)): end result = classify_image(body, PROMPTS) outcomes.append(end result) video_length = 10 * len(outcomes) video_length = video_length / 24
video_length = spherical(video_length, 2) print(f"The video is {video_length} seconds lengthy") timestamps = {} for i, end in enumerate(outcomes): closest_interval = int(i / INTERVAL_PERIOD) * INTERVAL_PERIOD if closest_interval not in timestamps: timestamps[closest_interval] = [result] else: timestamps[closest_interval].append(end result) for key, worth in timestamps.objects(): prev_key = max(0, key - INTERVAL_PERIOD) most_common = max(set(worth), key=worth.rely) print(f"From {prev_key} to {key + INTERVAL_PERIOD} seconds, the primary class is {most_common}")
On this code, we open our video file and, for every body, calculate essentially the most related label. We then calculate how lengthy our video is. Lastly, we group labels by interval (the INTERVAL_PERIOD
worth we set earlier).
For every interval (i.e. 0-5s, 5-10s, 10-15s), we discover the most typical label. We then assign that as a label for that timestamp.
Let’s run our script on the Contact trailer. Right here is an excerpt of the outcomes:
From Zero to five seconds, the primary class is satellite tv for pc
From Zero to 10 seconds, the primary class is satellite tv for pc
From 5 to 15 seconds, the primary class is satellite tv for pc
From 10 to 20 seconds, the primary class is satellite tv for pc
From 15 to 25 seconds, the primary class is satellite tv for pc
From 20 to 30 seconds, the primary class is satellite tv for pc
From 25 to 35 seconds, the primary class is satellite tv for pc
...
From 1970 to 1980 seconds, the primary class is satellite tv for pc
From 1975 to 1985 seconds, the primary class is one thing else
From 1980 to 1990 seconds, the primary class is one thing else
From 1985 to 1995 seconds, the primary class is laptop
From 1990 to 2000 seconds, the primary class is laptop
From 1995 to 2005 seconds, the primary class is laptop
...
Our script has efficiently assigned labels to completely different timestamps in our video.
We will course of the timestamps additional to know what proportion of a video matches every immediate. To take action, we are able to use this code:
percentage_of_video_prompt_coverage = {immediate: Zero for immediate in PROMPTS} for immediate in PROMPTS: counter = outcomes.rely(immediate) percentage_of_video_prompt_coverage[prompt] = counter / len(outcomes) for immediate, proportion in percentage_of_video_prompt_coverage.objects(): print(f"The immediate {immediate} is current in {spherical(proportion * 100, 2)}% of the video")
When analyzing the primary few seconds of our video, we get the breakdown:
The immediate satellite tv for pc is current in 30.31% of the video
The immediate workplace is current in 3.45% of the video
The immediate park is current in 0.0% of the video
The immediate laptop is current in 39.93% of the video
The immediate outside is current in 4.47% of the video
The immediate conferences is current in 0.67% of the video
The immediate one thing else is current in 21.16% of the video
With this logic, we are able to make determinations about our video.
For instance, if greater than 10% of a video matches “laptop”, we may classify the trailer with a label like “Expertise” in an inner media database. In a broadcasting situation, we might maintain any trailers that comprise violence till after a sure time of day, vital for complying with “watershed” laws the place violent supplies can’t be broadcast on air.
Utilizing CLIP and Gaudi2 for Video Classification
You should use CLIP to establish essentially the most related label to a body in a video. You should use this logic to categorise movies. You’ll be able to classify movies by timestamp. That is helpful for looking a video. For instance, you possibly can construct a search engine that permits you to search a particular video for a scene that matches a label like “laptop”.
To compute CLIP vectors for our software, we used a Gaudi2 chip. Gaudi2 is designed for high-performance AI workloads. The Transformers implementation of CLIP we used on this information is optimized to be used on Gaudi2.
You can use the logic we wrote on this information to index a big repository of movies in a batch. Or you possibly can construct a queue that classifies movies as they’re submitted to a system.
To study extra concerning the Gaudi2 system, confer with the Gaudi2 product reference on the Intel Habana web site.